Portrait enhancement
By using artificial intelligence technology to convert low resolution portraits into high-resolution portraits, the clarity and details of portraits can be improved.
If you've purchased, get your API KEY in personal center.
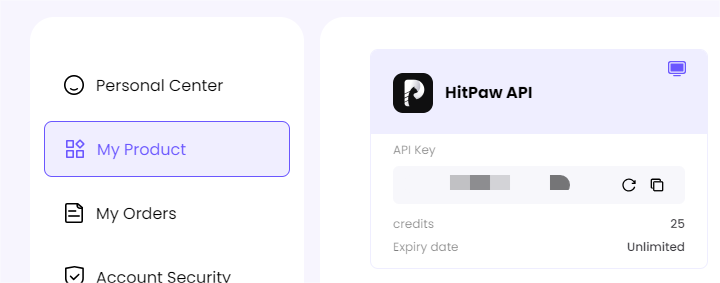
// Interface API for initiating photo-enhancer task requests
curl --location --request POST 'https://api.hitpaw.com/api/v3/photoEnhanceByUrl' \
--header 'APIKEY: cLr9XnMQFiEAK23YTHyx' \
--header 'Content-Type: application/json' \
--data-raw '{
"image_url":"https://piccutout-sgp-test.oss-accelerate.aliyuncs.com/static/2024-01-19/7ecaedcd-90cb-4921-9532-4991547d6103-1705633751000/input/7b4e41b42721b2ca706117223ecba6c7.png",
"image_format":".png"
}'
// Interface API for photo enhance task status result queries
curl --location --request POST 'https://api.hitpaw.com/api/v3/photo-enhance/status?job_id=nxcAZlh6yEatJeMUxbDbN4Aj' \
--header 'Content-Type: application/json' \
--data-raw '{
"job_id":"7ngh7A18FWPVZ2EEo3i0ovAW"
}'
// Task request interface API for initiating image object removal
curl --location --request POST 'https://api.hitpaw.com/api/v3/img-object-remove' \
--header 'APIKEY: cLr9XnMQFiEAK23YTHyx' \
--header 'User-Agent: Apifox/1.0.0 (https://apifox.com)' \
--header 'Content-Type: application/json' \
--data-raw '{
"image_url":"https://ai-hitpaw-us.oss-accelerate.aliyuncs.com/static/2024-01-19/330d8ade-a3e8-405d-bb9d-5c2323ded0ae-1705645200000/input/7b4e41b42721b2ca706117223ecba6c7.png",
"mask_url":"https://ai-hitpaw-us.oss-accelerate.aliyuncs.com/static/2024-01-19/f3ef87cf-14d8-4eff-9878-3be79bd16efb-1705645206000/input/38a79625c07d04ce368a04558be9c6d0.png"
}'
// The interface that initiates the request
import http.client
import json
conn = http.client.HTTPSConnection("api.hitpaw.com")
payload = json.dumps({
"image_url": "https://piccutout-sgp-test.oss-accelerate.aliyuncs.com/static/2024-01-19/7ecaedcd-90cb-4921-9532-4991547d6103-1705633751000/input/7b4e41b42721b2ca706117223ecba6c7.png",
"image_format": ".png"
})
headers = {
'APIKEY': 'cLr9XnMQFiEAK23YTHyx',
'User-Agent': 'Apifox/1.0.0 (https://apifox.com)',
'Content-Type': 'application/json'
}
conn.request("POST", "/api/v3/photoEnhanceByUrl", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
// Interface for querying task status results
import http.client
import json
conn = http.client.HTTPSConnection("https://api.hitpaw.com")
payload = json.dumps({
"job_id": "7ngh7A18FWPVZ2EEo3i0ovAW"
})
headers = {
'User-Agent': 'Apifox/1.0.0 (https://apifox.com)',
'Content-Type': 'application/json'
}
conn.request("POST", "/api/v3/photo-enhance/status?job_id=nxcAZlh6yEatJeMUxbDbN4Aj", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
// The interface that initiates the request
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.hitpaw.com/api/v3/photoEnhanceByUrl');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'APIKEY' => 'cLr9XnMQFiEAK23YTHyx',
'User-Agent' => 'Apifox/1.0.0 (https://apifox.com)',
'Content-Type' => 'application/json'
));
$request->setBody('{\n "image_url":"https://piccutout-sgp-test.oss-accelerate.aliyuncs.com/static/2024-01-19/7ecaedcd-90cb-4921-9532-4991547d6103-1705633751000/input/7b4e41b42721b2ca706117223ecba6c7.png",\n "image_format":".png"\n}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
// Interface for task query
<?php
require_once 'HTTP/Request2.php';
$request = new HTTP_Request2();
$request->setUrl('https://api.hitpaw.com/api/v3/photo-enhance/status?job_id=nxcAZlh6yEatJeMUxbDbN4Aj');
$request->setMethod(HTTP_Request2::METHOD_POST);
$request->setConfig(array(
'follow_redirects' => TRUE
));
$request->setHeader(array(
'User-Agent' => 'Apifox/1.0.0 (https://apifox.com)',
'Content-Type' => 'application/json'
));
$request->setBody('{\n "job_id":"7ngh7A18FWPVZ2EEo3i0ovAW"\n}');
try {
$response = $request->send();
if ($response->getStatus() == 200) {
echo $response->getBody();
}
else {
echo 'Unexpected HTTP status: ' . $response->getStatus() . ' ' .
$response->getReasonPhrase();
}
}
catch(HTTP_Request2_Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
// Interface for initiating task requests
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"image_url\":\"https://piccutout-sgp-test.oss-accelerate.aliyuncs.com/static/2024-01-19/7ecaedcd-90cb-4921-9532-4991547d6103-1705633751000/input/7b4e41b42721b2ca706117223ecba6c7.png\",\n \"image_format\":\".png\"\n}");
Request request = new Request.Builder()
.url("https://api.hitpaw.com/api/v3/photoEnhanceByUrl")
.method("POST", body)
.addHeader("APIKEY", "cLr9XnMQFiEAK23YTHyx")
.addHeader("User-Agent", "Apifox/1.0.0 (https://apifox.com)")
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
// Interface for task query
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"job_id\":\"7ngh7A18FWPVZ2EEo3i0ovAW\"\n}");
Request request = new Request.Builder()
.url("https://api.hitpaw.com/api/v3/photo-enhance/status?job_id=nxcAZlh6yEatJeMUxbDbN4Aj")
.method("POST", body)
.addHeader("User-Agent", "Apifox/1.0.0 (https://apifox.com)")
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
// Interface for initiating task requests
var myHeaders = new Headers();
myHeaders.append("APIKEY", "cLr9XnMQFiEAK23YTHyx");
myHeaders.append("User-Agent", "Apifox/1.0.0 (https://apifox.com)");
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"image_url": "https://piccutout-sgp-test.oss-accelerate.aliyuncs.com/static/2024-01-19/7ecaedcd-90cb-4921-9532-4991547d6103-1705633751000/input/7b4e41b42721b2ca706117223ecba6c7.png",
"image_format": ".png"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.hitpaw.com/api/v3/photoEnhanceByUrl", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
// Interface for task query
var myHeaders = new Headers();
myHeaders.append("User-Agent", "Apifox/1.0.0 (https://apifox.com)");
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"job_id": "7ngh7A18FWPVZ2EEo3i0ovAW"
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.hitpaw.com/api/v3/photo-enhance/status?job_id=nxcAZlh6yEatJeMUxbDbN4Aj", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
// Interface for initiating task requests
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.hitpaw.com/api/v3/photoEnhanceByUrl"
method := "POST"
payload := strings.NewReader(`{
"image_url":"https://piccutout-sgp-test.oss-accelerate.aliyuncs.com/static/2024-01-19/7ecaedcd-90cb-4921-9532-4991547d6103-1705633751000/input/7b4e41b42721b2ca706117223ecba6c7.png",
"image_format":".png"
}`)
client := &http.Client {
}
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("APIKEY", "cLr9XnMQFiEAK23YTHyx")
req.Header.Add("User-Agent", "Apifox/1.0.0 (https://apifox.com)")
req.Header.Add("Content-Type", "application/json")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
// Interface for task query
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.hitpaw.com/api/v3/photo-enhance/status?job_id=nxcAZlh6yEatJeMUxbDbN4Aj"
method := "POST"
payload := strings.NewReader(`{
"job_id":"7ngh7A18FWPVZ2EEo3i0ovAW"
}`)
client := &http.Client {
}
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("User-Agent", "Apifox/1.0.0 (https://apifox.com)")
req.Header.Add("Content-Type", "application/json")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
API documentation description
Request Description
- Request URL: https://api.hitpaw.com/api/v3/photoEnhanceByUrl
- Request method: POST
- Return type: job_id
- input parameters:
-
(1) Request Parameters (Header)
parameter Parameter type describe describe describe APIKEY APIKEY Java Java Java -
(2) Request parameters (Body)
{ "image_url":"https://piccutout-sgp-test.oss-accelerate.aliyuncs.com/static/2024-01-19/7ecaedcd-90cb-4921-9532-4991547d6103-1705633751000/input/7b4e41b42721b2ca706117223ecba6c7.png", // imageurl "image_format":".png" // Desired output image format }
Return instructions
Return parameters
{
"code": 200,
"data": {
"job_id": "tZgnWAwQT80RgLNp4QToNHSW" //The current task id, used in the task query interface job_id parameter.
},
"message": "OK"
}
Price Description
You can purchase API call points through online recharge and payment methods
Frequently Asked Questions
Q: What are the requirements for the format of the input image?
A: Supports PNG, JPG, JPEG, BMP, TIFF, but currently does not support GIF type animations.
Q: Is there a size limit for supporting images?
A: At present, the maximum resolution uploaded is 4096x4096 pixels, and the image file size is below 20MB.